Hackerrank - Strings: Making Anagrams Solution
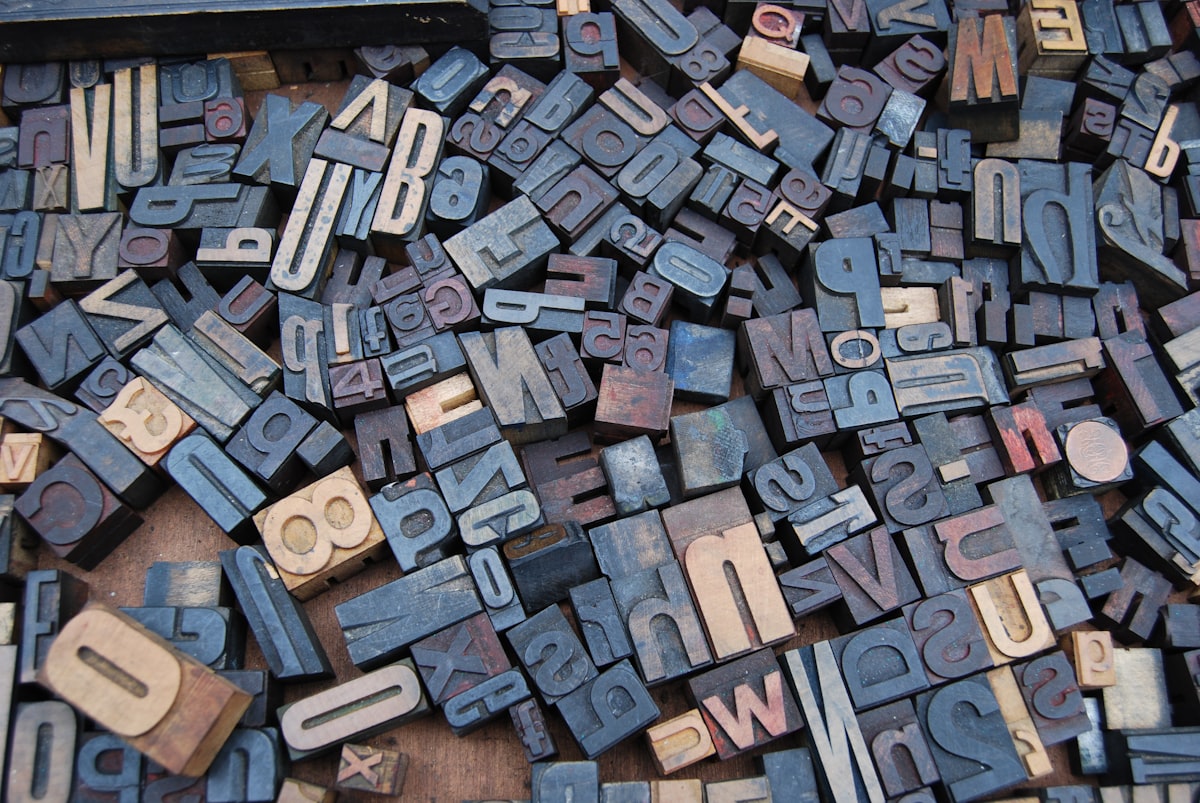
Alice is taking a cryptography class and finding anagrams to be very useful. We consider two strings to be anagrams of each other if the first string's letters can be rearranged to form the second string. In other words, both strings must contain the same exact letters in the same exact frequency For example, bacdc
and dcbac
are anagrams, but bacdc
and dcbad
are not.
Alice decides on an encryption scheme involving two large strings where encryption is dependent on the minimum number of character deletions required to make the two strings anagrams. Can you help her find this number?
Given two strings, and , that may or may not be of the same length, determine the minimum number of character deletions required to make and anagrams. Any characters can be deleted from either of the strings.
For example, if and , we can delete from string and from string so that both remaining strings are and which are anagrams.
Function Description
Complete the makeAnagram function in the editor below. It must return an integer representing the minimum total characters that must be deleted to make the strings anagrams.
makeAnagram has the following parameter(s):
- a: a string
- b: a string
Input Format
The first line contains a single string, .
The second line contains a single string, .
Constraints
- The strings and consist of lowercase English alphabetic letters ascii[a-z].
Output Format
Print a single integer denoting the number of characters you must delete to make the two strings anagrams of each other.
Sample Input
cde
abc
Sample Output
4
Explanation
We delete the following characters from our two strings to turn them into anagrams of each other:
- Remove
d
ande
fromcde
to getc
. - Remove
a
andb
fromabc
to getc
.
We must delete characters to make both strings anagrams, so we print on a new line.
Solution in Python
Solution 1
from collections import Counter
def makeAnagram(a, b):
return len(a)+len(b)-sum((Counter(a) & Counter(b)).values())*2
a = input()
b = input()
print(makeAnagram(a, b))
Solution 2
from collections import Counter
def makeAnagram(a, b):
a = Counter(a)
b = Counter(b)
return sum(((a | b) - (a & b)).values())
a = input()
b = input()
print(makeAnagram(a, b))